Creating a VR baseball game in Unity is easier than you think. With some patience and practice, you can build an exciting game.
Virtual reality (VR) has changed how we experience games. It brings us right into the action. Unity is a powerful tool for making VR games. Even beginners can use it. This guide will show you the steps to make a VR baseball game.
You’ll learn the basics of Unity and some key features. You’ll also see how to set up the VR environment. By the end, you’ll have a fun game to share. Get ready to dive into the world of VR and game development!
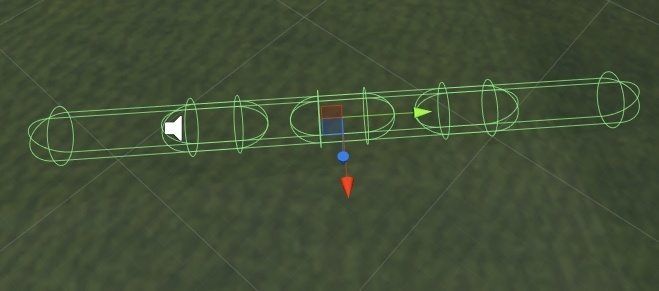
Credit: unity3d.college
Introduction To Vr Baseball
Virtual Reality (VR) is changing the way we experience games. VR Baseball is one such game that offers an immersive and exciting experience. Imagine stepping into a virtual ballpark where you can hit home runs or pitch fastballs. This is the magic of VR Baseball.
Why Choose Vr
Choosing VR for a baseball game brings many advantages. It provides a realistic experience that traditional games can’t match. Players feel like they are actually on the field. This level of immersion makes the game more engaging.
Another reason to choose VR is the interactive environment. Players can interact with the game in ways that are not possible with regular games. They can use their body movements to swing the bat or throw the ball.
Benefits Of Vr In Sports Games
There are many benefits of using VR in sports games. Here are some key points:
- Enhanced Learning: VR helps players learn the game better. They can practice their skills in a controlled environment.
- Physical Activity: Playing VR games involves physical movement. This can be a fun way to stay active.
- Realistic Training: Athletes can use VR for training. They can simulate game situations and improve their performance.
- Immersive Experience: VR provides a deep sense of immersion. Players feel like they are part of the game.
These benefits make VR an excellent choice for sports games like baseball. It offers a unique and engaging experience that players will love.
Setting Up Unity
Setting up Unity is a crucial step in creating your VR baseball game. It involves installing the necessary software and configuring it for VR. Proper setup ensures that your game runs smoothly and offers a great experience for players.
Installing Unity
First, download the Unity Hub from the official Unity website. The Unity Hub helps you manage different versions of Unity. Install the Hub and open it to proceed.
Next, click on the “Installs” tab and then “Add” to select the Unity version. Choose the latest LTS (Long Term Support) release for stability. Follow the prompts to complete the installation process.
After installation, create a new project by clicking on the “Projects” tab. Select “New Project” and choose the 3D template. Name your project and choose the location to save it. Click “Create” to open your new Unity project.
Configuring Unity For Vr
To configure Unity for VR, you need to install the XR Plugin Management package. Open the Unity Editor, go to “Window” and select “Package Manager.” In the Package Manager, search for “XR Plugin Management” and install it.
Once installed, go to “Edit” and select “Project Settings.” Under the XR Plugin Management tab, enable the VR SDKs you plan to use. Popular options include Oculus and OpenVR. Make sure to install these SDKs if you haven’t already.
Configure your project settings to optimize for VR. Go to “Edit” and select “Project Settings.” Under “Player,” set the rendering path to “Forward” for better performance. Also, enable “Multithreaded Rendering” for smoother gameplay.
Finally, test your VR setup by connecting your VR headset. Ensure the headset is recognized and working correctly. Your Unity project is now ready for VR development.
Creating The Game Environment
Creating the game environment for your VR baseball game in Unity is a crucial step. This sets the stage for player immersion. A well-designed environment enhances gameplay and boosts user engagement. Let’s dive into the key aspects of this process.
Designing The Baseball Field
The baseball field is the heart of your VR game. Start by creating a base terrain. Use Unity’s terrain tools to sculpt the landscape. Ensure the field has a flat, smooth surface. This makes the game more realistic.
- Open Unity and create a new project.
- Go to the Terrain tab and select Create Terrain.
- Sculpt the terrain to form a flat baseball field.
Next, add the baseball diamond. This includes the infield and outfield. Mark the bases and pitcher’s mound clearly. Use textures to differentiate between grass, dirt, and base paths.
- Import textures for grass, dirt, and base paths.
- Apply these textures to the appropriate areas of the field.
- Place objects to mark the bases and pitcher’s mound.
Adding 3d Models
3D models bring your baseball field to life. You’ll need models for bases, bleachers, and dugouts. Download or create these models.
Model | Purpose |
---|---|
Bases | Mark base positions |
Bleachers | Provide seating for spectators |
Dugouts | Designate player areas |
Import these models into Unity. Place them in the correct spots on the field. Ensure they are scaled correctly to match the player’s perspective.
- Download or create 3D models for the field.
- Import these models into Unity.
- Place and scale them appropriately.
Adding details like trees, scoreboards, and fences enhances realism. These elements add depth to the game environment. Arrange them thoughtfully to create a cohesive look.
Follow these steps to create an immersive baseball field. Design the environment carefully. Use the right models to enhance the game’s realism.
Implementing Player Controls
Implementing player controls in a VR baseball game is crucial. It enhances the player experience and makes the game more immersive. In this section, we will cover how to set up motion controls and swing mechanics in Unity. These steps will help you create a realistic and engaging VR baseball game.
Motion Controls Setup
First, you need to set up motion controls. This allows players to move their hands and interact with the game. Unity supports various VR headsets like Oculus Rift and HTC Vive. Make sure your VR headset is compatible.
Here’s a simple setup guide:
- Install the latest Unity version.
- Set up your VR headset and controllers.
- Open Unity and create a new project.
- Go to Window > Package Manager and install the XR Plugin Management package.
- Configure the XR settings in the Project Settings.
Next, you need to add VR support to your game objects. Attach VR controller scripts to the player’s hands. This lets the game recognize hand movements.
Swing Mechanics
Swing mechanics make the game feel real. To implement swing mechanics, follow these steps:
- Create a bat model in Unity.
- Attach the bat to the player’s hand.
- Write a script to detect swing motion.
- Use the VR controller’s velocity to determine the swing speed.
- Play a sound effect when the bat hits the ball.
Here’s a simple script to detect a swing:
using UnityEngine;
public class SwingMechanics : MonoBehaviour
{
public AudioSource swingSound;
void Update()
{
if (Input.GetButtonDown("Fire1"))
{
SwingBat();
}
}
void SwingBat()
{
// Add swing logic here
swingSound.Play();
}
}
This script plays a sound when the player swings the bat. You can expand this script to include more complex swing mechanics.
Building Game Mechanics
Creating a VR baseball game in Unity requires strong game mechanics. The mechanics ensure smooth and realistic gameplay. This section will focus on key components. We will cover Pitching Logic and Batting Logic.
Pitching Logic
Pitching is a core part of the game. To start, create a script for the pitcher. This script will control how the ball is thrown. Use Unity’s physics engine to add realism.
- Determine the speed and angle of the pitch.
- Randomize these values to simulate different pitch types.
- Incorporate a pitching animation for visual effect.
Here is a basic code snippet for pitching:
public class Pitch : MonoBehaviour {
public float pitchSpeed = 10f;
public Transform pitchPoint;
void Start() {
Rigidbody rb = GetComponent();
rb.velocity = pitchPoint.forward pitchSpeed;
}
}
This script makes the ball move forward from the pitch point. Adjust pitchSpeed
for varied pitches.
Batting Logic
Next is batting. Batting involves user interaction. Create a script for the batter. This script will detect swing actions and contact with the ball.
- Track the position of the bat.
- Detect collisions between the bat and the ball.
- Determine the force and direction of the hit.
Here is a sample code for the batting mechanism:
public class Bat : MonoBehaviour {
public Transform batTip;
public float swingForce = 15f;
void OnTriggerEnter(Collider other) {
if (other.CompareTag("Ball")) {
Rigidbody rb = other.GetComponent();
Vector3 hitDirection = batTip.forward;
rb.velocity = hitDirection swingForce;
}
}
}
This script applies force to the ball when the bat hits it. Adjust swingForce
for more powerful hits.
By implementing these mechanics, you can create a realistic VR baseball game. Experiment with different values to fine-tune your game.
Integrating Vr Features
Integrating VR features into your Unity baseball game can make it more immersive and interactive. This section will guide you through some key aspects of integrating VR features, including headset compatibility and hand tracking.
Headset Compatibility
Ensuring your VR baseball game supports different headsets is crucial. Unity provides tools to make this easier. You can use the XR Plugin Management system in Unity. This system helps you manage multiple VR devices.
Follow these steps to set up headset compatibility:
- Open Unity and go to the Project Settings menu.
- Select XR Plugin Management.
- Enable the plugins for the headsets you want to support, such as Oculus and HTC Vive.
- Test your game on each headset to ensure it works correctly.
By following these steps, your game will be playable on multiple VR headsets.
Hand Tracking
Adding hand tracking to your VR baseball game enhances player interaction. Hand tracking allows players to use their hands naturally instead of controllers.
To integrate hand tracking, use the Oculus Integration package in Unity. Here’s how to do it:
- Download the Oculus Integration package from the Unity Asset Store.
- Import the package into your Unity project.
- Set up hand tracking by following the Oculus documentation.
- Test the hand tracking features to ensure they work well in your game.
With hand tracking, players can swing bats and catch balls using their hands. This makes the game feel more realistic.
Integrating these VR features will enhance your baseball game. Players will have a more immersive and enjoyable experience.
Testing And Debugging
Testing and debugging are essential steps in developing a VR baseball game in Unity. These stages help identify and fix problems, ensuring a smooth gaming experience. Let’s explore some common issues and how to optimize performance.
Common Issues
Here are some common issues you might face:
- Tracking Problems: The VR headset may lose track of the player. Make sure sensors are properly positioned.
- Lag and Stuttering: This can disrupt gameplay. Ensure your code is optimized.
- Collision Detection: Objects may pass through each other. Check your collision settings.
Optimizing Performance
Optimizing performance is crucial for a smooth VR experience. Here are some tips:
- Reduce Polygon Count: Use simpler models to improve performance.
- Use LOD (Level of Detail): Apply LOD to reduce detail on distant objects.
- Optimize Code: Ensure your scripts are efficient and avoid unnecessary calculations.
- Use Occlusion Culling: This technique hides objects not currently visible to the player.
Below is a table summarizing common issues and their solutions:
Issue | Solution |
---|---|
Tracking Problems | Adjust sensor positions |
Lag and Stuttering | Optimize code |
Collision Detection | Check collision settings |
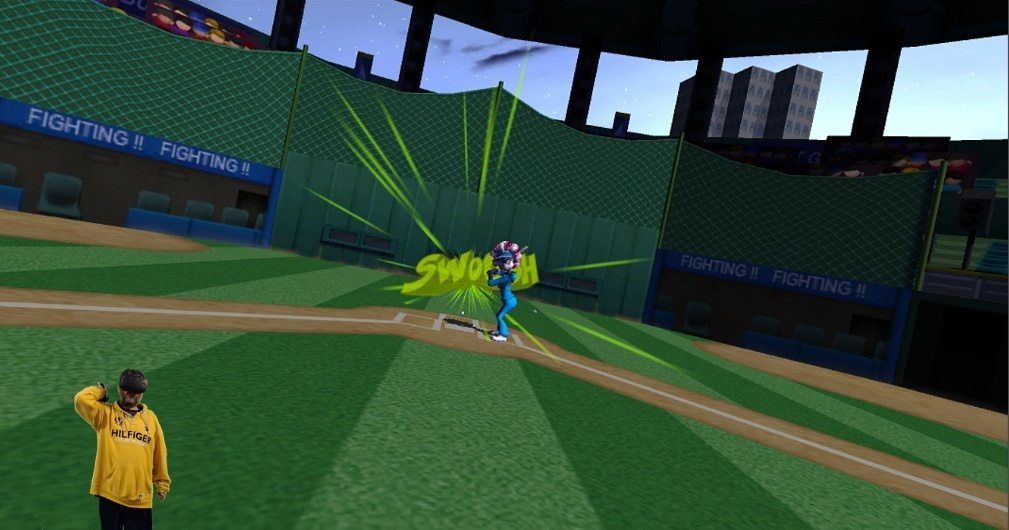
Credit: unity3d.college
Publishing Your Game
Publishing your VR baseball game can be an exciting milestone. This step involves several key tasks. It’s not just about making the game available but also ensuring it reaches the right audience. Let’s break it down into manageable sections.
Preparing For Release
Before releasing your VR baseball game, verify that it meets quality standards. Perform extensive testing to identify and fix bugs. Ensure the game runs smoothly on different devices. Gather feedback from beta testers and make necessary adjustments.
Next, prepare your game for submission to various platforms. Create a compelling game description. Include high-quality screenshots and engaging videos. These elements help attract potential players.
Task | Details |
---|---|
Testing | Identify and fix bugs, ensure smooth performance. |
Feedback | Gather from beta testers, make adjustments. |
Submission | Create descriptions, include screenshots and videos. |
Marketing Strategies
Marketing your VR baseball game effectively is crucial. Start by identifying your target audience. Create a marketing plan that outlines your goals and strategies.
Use social media to build buzz around your game. Share behind-the-scenes content, teaser trailers, and updates. Engage with your audience by responding to comments and messages.
Consider collaborating with influencers in the gaming community. They can help promote your game to a wider audience. Additionally, launch a press kit to distribute to gaming websites and blogs. This kit should include details about your game, along with high-quality images and videos.
- Identify target audience
- Create a marketing plan
- Use social media
- Collaborate with influencers
- Launch a press kit
Measure the success of your marketing efforts. Use analytics tools to track engagement and downloads. Adjust your strategies based on the data collected.

Credit: www.youtube.com
Frequently Asked Questions
How Do I Start A Vr Baseball Game In Unity?
To start, install Unity and create a new project. Import necessary assets and set up a basic scene.
What Assets Are Needed For A Vr Baseball Game?
You’ll need player models, baseball field assets, VR controllers, and sound effects. Ensure compatibility with VR.
How Do I Implement Vr Controls In Unity?
Use Unity’s XR Interaction Toolkit. Configure the controllers to interact with the game objects for an immersive experience.
Can I Use Pre-made Animations For My Vr Baseball Game?
Yes, you can use pre-made animations. Import them into Unity and apply them to your player models.
Conclusion
Creating a VR baseball game in Unity can be rewarding. Start small. Learn each step well. Use simple code and clear assets. Test often. Make improvements as needed. Your game will get better with practice. Enjoy the process. Share your game with friends.
Get feedback. Keep learning and improving. Have fun developing your VR baseball game!